27/march/2021
Things you cannot do
•You cannot
- use “=” to assign one array variable to another:
a = b; /* a and b are arrays */
-use “==” to directly compare array variables:
if (a==b) .……… . .
- directly scanf or printf arrays:
printf (“……”, a) ;
- The size may be omitted. In such cases, the compiler automatically allocates enough space for all initialized elements.
int flag [] = {1, 1, 1,0} ;
char name [] = {‘A’, ‘m’, ‘i’ , ‘t’ } ;
How to copy the elements of one array to another?
• By copying individual elements:
How to read the elements of an array?
• The elements can be entered all in one line or different lines.
How the print the elements of an array?
• By printing them one element at a time.
• The elements are printed one per line.
Character string
Introduction
• A string is an array of characters.
-Individual characters are stored in memory in ASCII code.
- A string is represented as a sequence of characters terminated by the null (‘\0’) character.
Declaring string variable
• A string is declared like any other array:
char string-name [size] ;
-size determines the number of characters in
string_name.
• When a character string is assigned to a character
array, it automatically appends the null character
(‘\0’) at the end of the string.
-Size should be equal to the number of characters
in the string plus one.
Examples
char name [30] ;
char city [15];
char dob [11];
• A string may be initialized at the time of
declaration.
Reading “words”
• scanf can be used with the “%s” format specification.
Char name [30] ;
:
:
Scanf (“%s”, name) ;
- The ampersand (&) is not required before the variable name with “%s”.
- The problem here is that the string is taken to be up to the first white space (blank, tab, carriage return, etc.)
- If we type “Rupak Biswas”
- Name will be assigned the string “Rupak”
Reading a “line of text”
• In many applications, we need to read in the entire line of text (including blank spaces).
• We can use the getchar() function for the purpose.
Writing strings to the screen
• We can use printf with the “%s” format
specification.
Processing character strings
• There exists a set of C library functions for
character string manipulation.
- strcpy :: string copy
- strlen :: string length
- strcmp :: string comparison
• It is required to add the line
#include <string.h>
Writing string to the screen
• We can use printf with the “%s” format
specification.
25/march/2021
Basic concept
• Many applications require multiple data items
that have common characteristics
• In mathematics, we often express such groups of
data items in indexed form:
x1,x2,x3…..,xn
• Why are arrays essential for some applications?
- Take an example.
- Finding the minimum of a set of numbers.
Using Arrays
• All the data items constituting the group share
the same name.
Int x[10];
• Individual elements are accessed by specifying
the index.
Declaring Arrays
• Like variables, the arrays that are used in a
program must be declared below they are used.
• General syntax:
type array-name [size];
- type specifies the type of element that will be
contained in the array (int, float, char, etc.).
- size is an integer constant that indicates the
maximum number of elements that can be
stored.
• Example
int x[10];
char line[80];
float points[150];
char name [35];
• If we are not sure of the exact size of the array, we
can define an array of a large size.
int marks [50];
char line [80];
float points[150];
char name[35];
• If we are not sure of the exact size of the array,
we can define an array of a large size.
int marks[50];
though in a particular run we may only be using
say, 10 elements.
• Starting from a given memory location, the
successive array elements are allocated space in
consecutive memory locations.
Array a
x x+k x+2k
X: starting address of the array in memory
K: number of bytes allocated per array element
• element a[i]:: allocated memory at the address
x+ i*k
• First array index assumed to be at zero.
Accessing Array Elements
• A particular of the array can be accessed by
specifying two things:
- Name the array.
- Index (relative position) of the element in the
array.
• In C, the index of an array starts from zero.
• Example:
- An array is defined as int x[10];
- The first element of the array x can be
accessed as x[0], the fourth element as x[3],
tenth element as x[9], etc.
Contd.
• The array index must evaluate to an
integer between 0 and n-1 where n is the
number of elements in the array.
a[x+2] = 25;
b[3*x-y] = a[10-x]+5;
A Warning
• In C, while accessing array elements,
array bounds are not checked.
• Example:
int marks[5];
• Example:
int marks[5];
:
:
marks[8] = 75
- The above assignment would not necessarily
cause an error.
- Rather, it may result in unpredictable
program results.
Initialization of Arrays
• General form:
type array_name [size] = { list of values } ;
• Examples:
int marks [5] = {72, 83, 65, 80, 76};
char name [4]= {‘A’, ‘m’, ‘i’, ‘t’ };
• Some special cases:
- if the number of values in the list is less than
the number of elements, the remaining
elements are automatically set to zero.
float total [5] = {24.2, -12.5, 35.1};
total [0] = 24.2, total [1] =-12.5, total [2] =
35.1,
total [3] = 0, total [4] = 0
Contd.
-The size may be omitted. In such cases, the
compiler automatically allocates enough
space for all initialized elements.
Int flag [ ] = {1, 1,1,0};
char name[ ] = { ‘A’, ‘m’, ‘i’, ‘t’, };
Example 1: Find the minimum of a set of 10
numbers
Alternate
Version 1
# include < stdio.h >
# include < conio.h>
Main (void )
{
int a,b,c;
printf (“sum-1 \n, sub-2\n, multi-3\n, div-4\n, mod-5\n”);
printf (“Enter the choice : \n”);
scanf (“%d “ ,&a,&b);
If (c==2)
Sub = a-b;
Else if (c==3)
Multiply =a*b;
Else if (c ==4)
Divide =a/b;
Else if (c == 5)
Mod = a%b;
Getch();
}
• We can use any level of nesting with the switch
case statement.
• Default:- When no case is matched, at that
level default is executed.
• We can use any loop inside the switch case statement.
How we can use char data with switch case
# include(stdio.h)
//used to clear input buffer.
Scanf (“/c” , &c );
Switch (c)
{
case ‘a’;
case ‘A’;
case ‘t’;
x = a+b;
break;
• We enter in switch case which we want to
check
Case ‘s’;
Case ‘r’;
case ‘*’;
x= a*b,
break;
case ‘d’;
case ’D’;
case ‘I’;
switch (b)
{
case o : printf ( “wrong data”);
printf ( “Denominator is zero”);
printf ( “Enter non zero value for
denominator”);
scanf (“%d” ,&b );
x=a/b;
break;
default,
x = a/b;
}
break;
default
{
printf ( “result = %d”,x);
ProgramsC programming initialsConstant/ Variable/KeywordLecture 10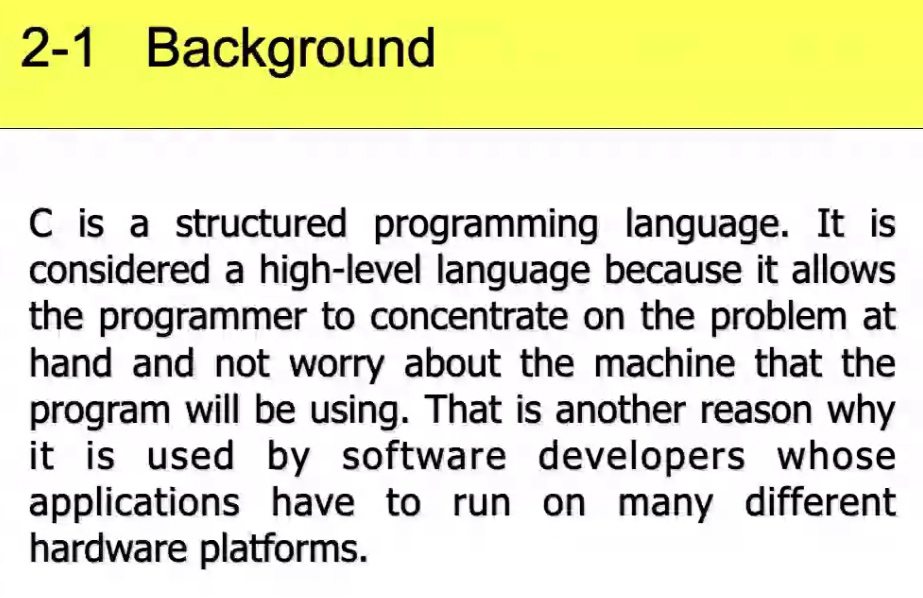
Lecture 7Lecture 6Lecture 54th Lecture3rd lecture2nd lecture
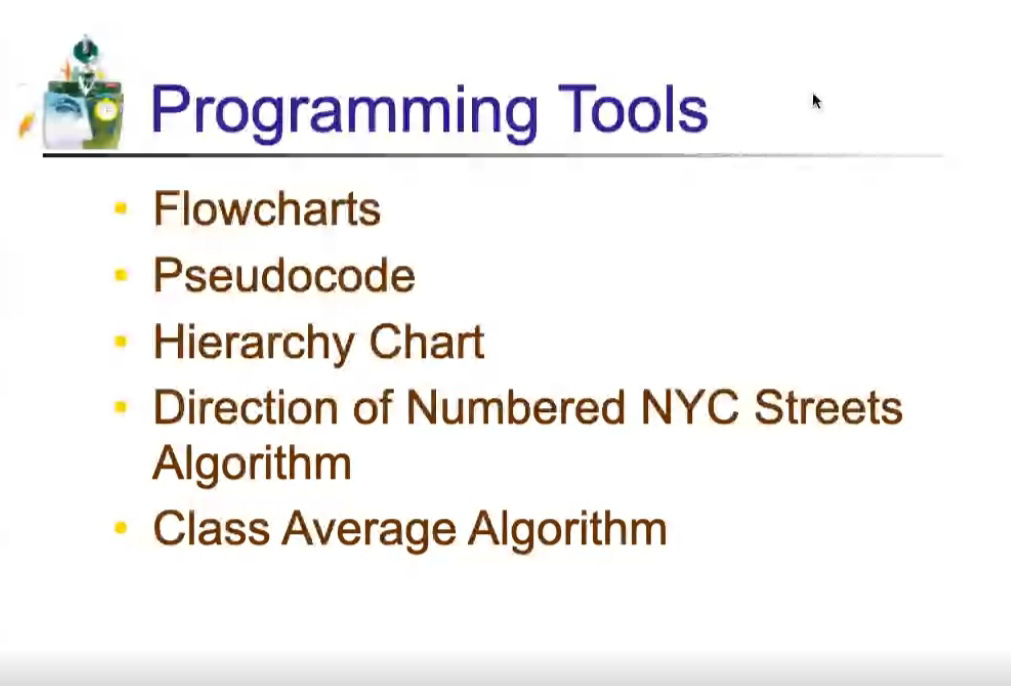
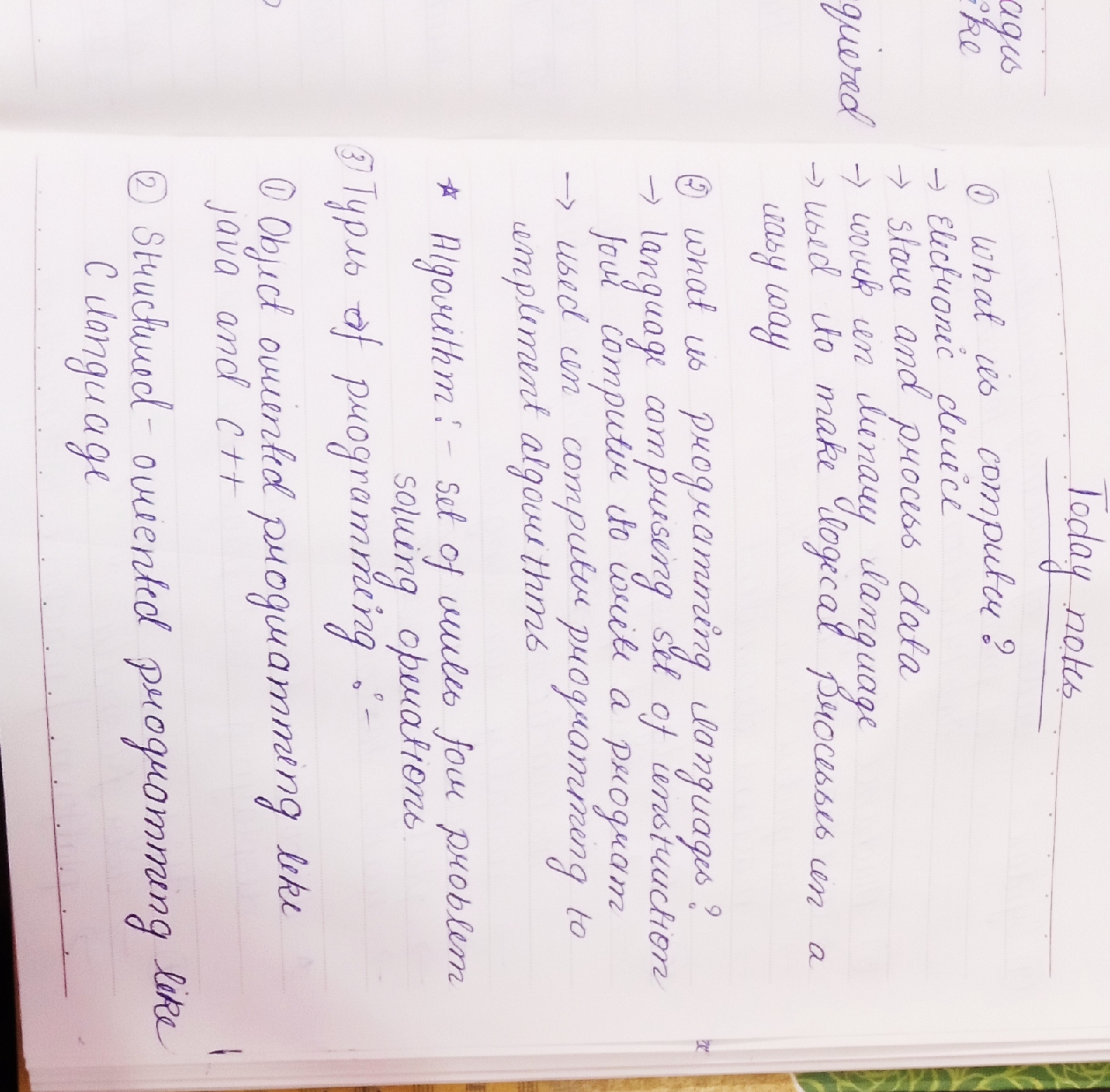
Comments
Post a Comment